iFrame
Introduction
Eagle Eye Networks provides different options to integrate live and recorded videos into your applications. They are as follows:
- Live video SDK
- Live video on web and Recorded video on web via API
- iFrame, representative of the live view and history browser view of the Eagle Eye Cloud VMS web application.
In this guide, we are going to demonstrate how to implement the iFrame feature for the Live Player and History Browser in the Eagle Eye web application.
Before you begin
- Login to your account and receive an access token.
- Using the /cameras API, retrieve a list of the connected cameras to the end user.
- Select the camera ID that you want to show in the iFrame.
Procedure
- Select the desired iFrame feature, and replace
<esn>
with your camera ID:
- In the Live Player:
https://iframe.eagleeyenetworks.com/#/live/<esn>
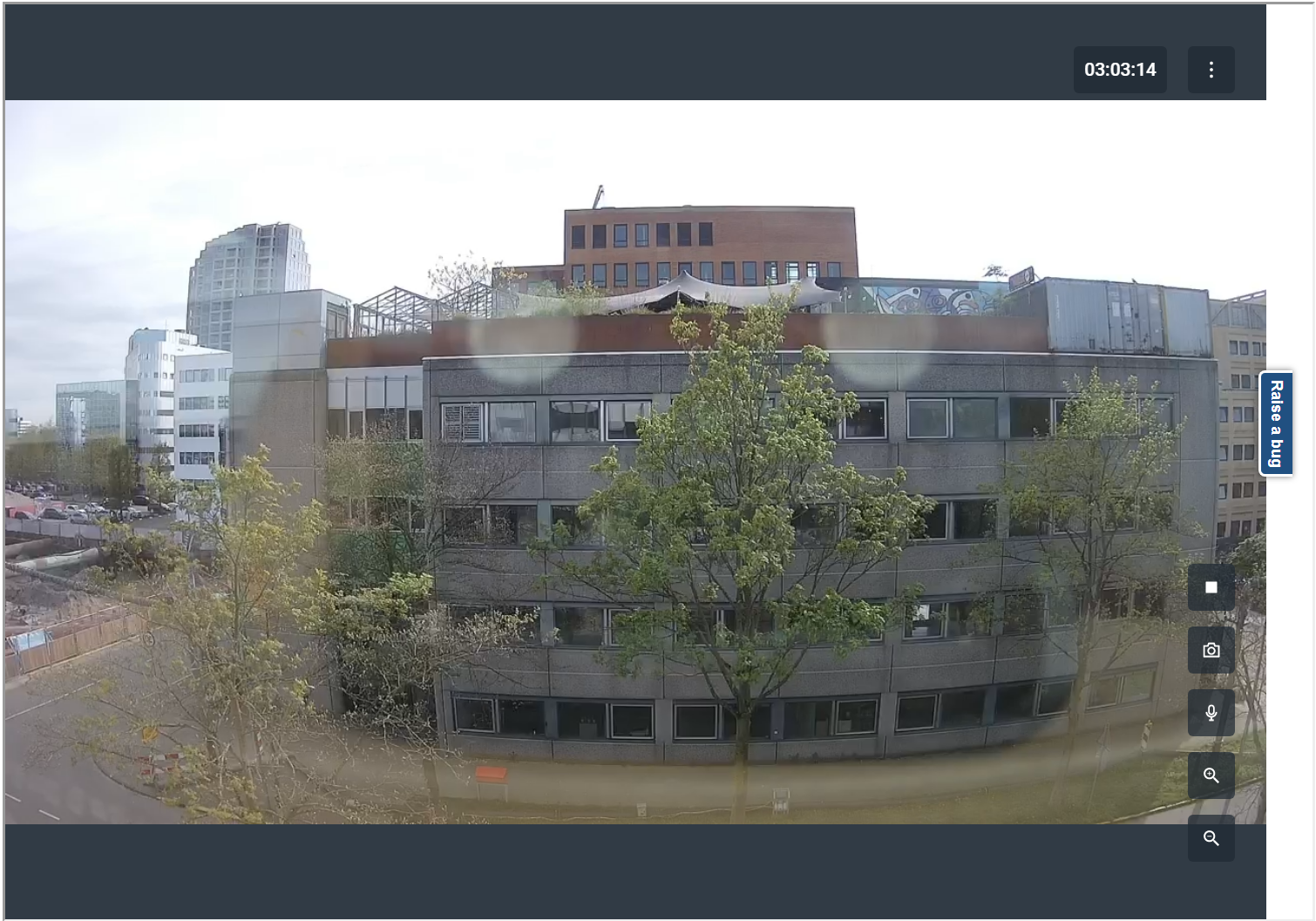
- In the History Browser:
https://iframe.eagleeyenetworks.com/#/history?ids=<esn>&time=<time_stamp>
(The time query parameter is optional)
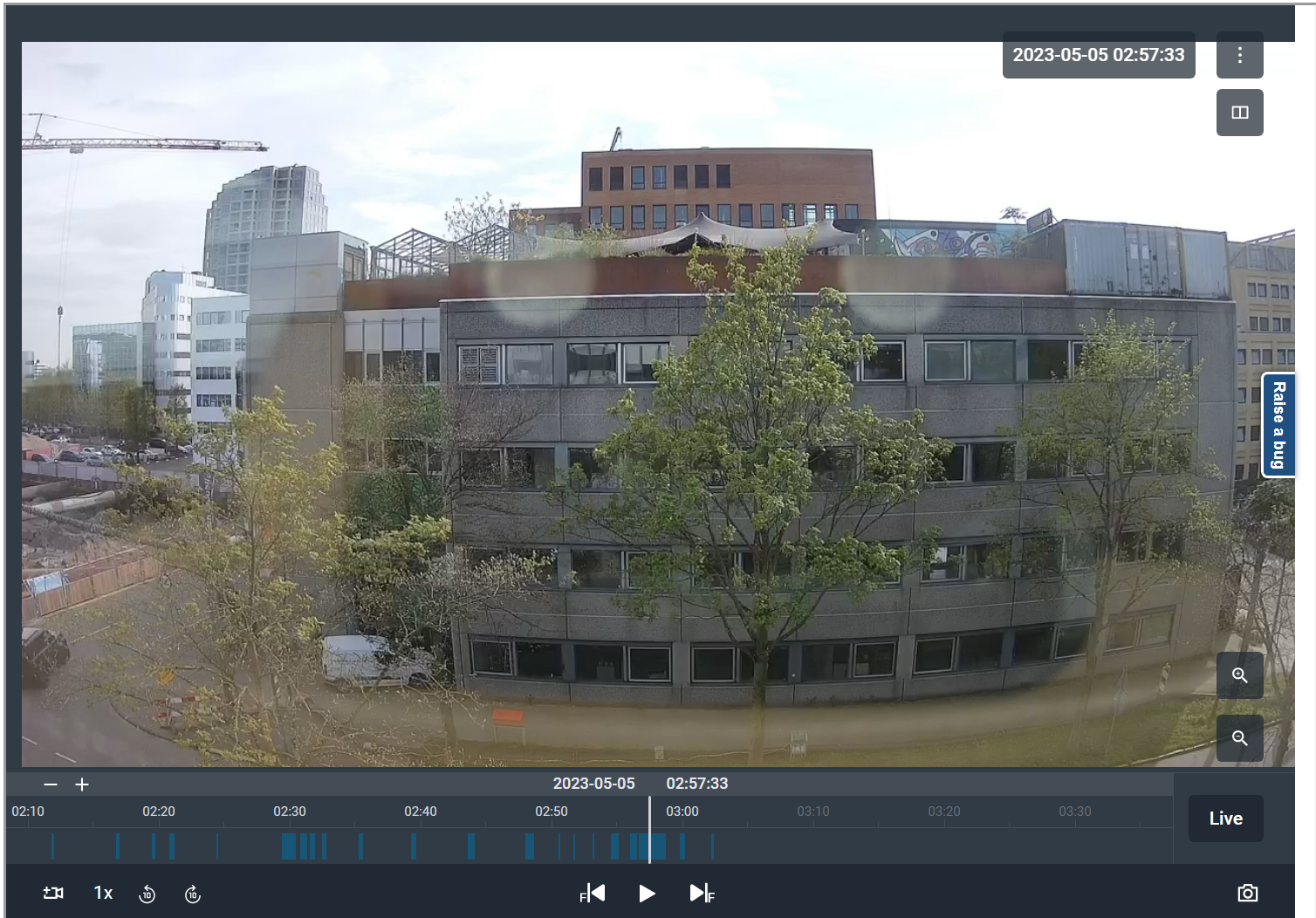
- Live + History:
https://iframe.eagleeyenetworks.com/#/historylive/<esn>
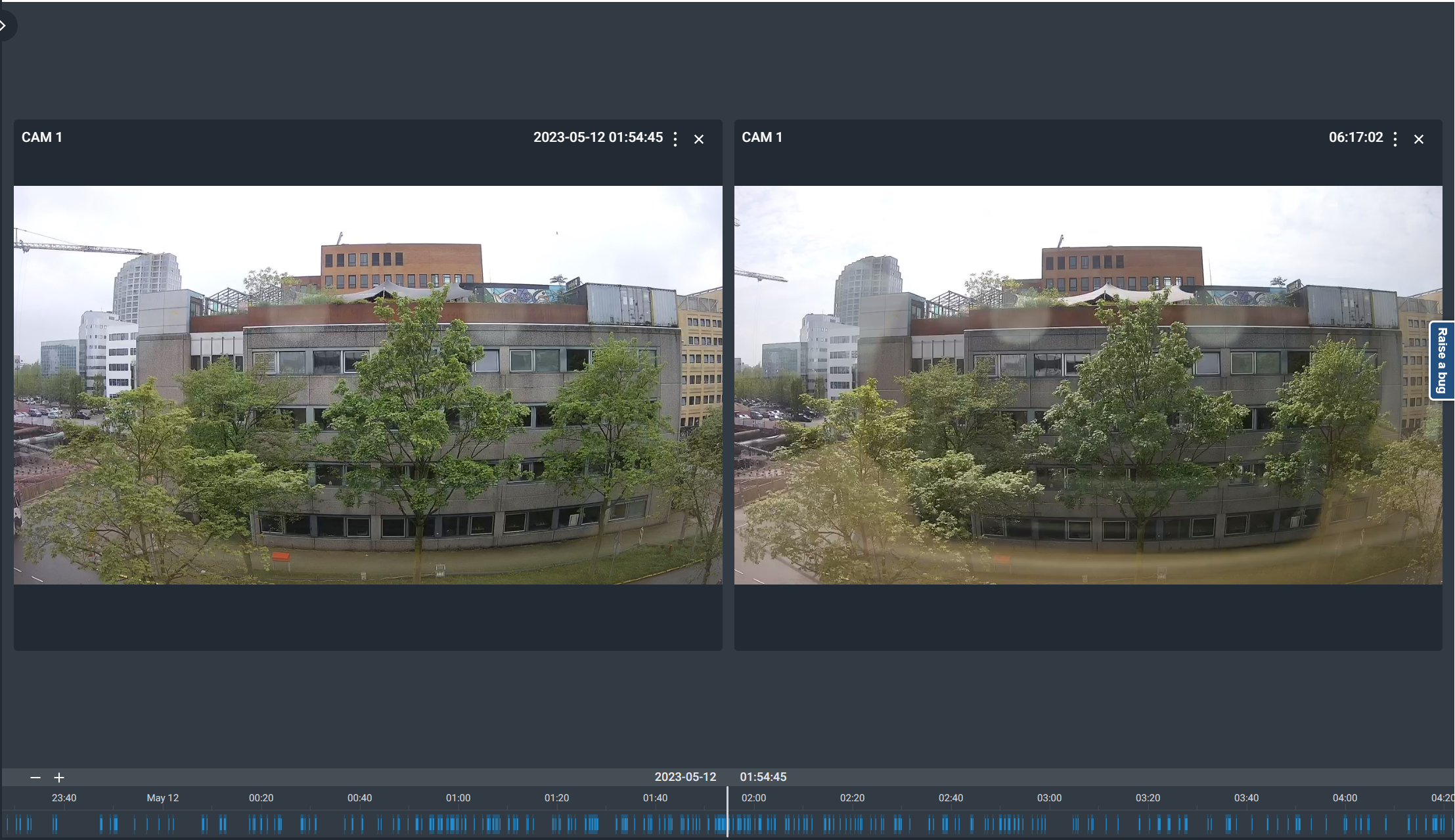
- Insert an iFrame element in the body and define the following attributes:
- id:
<string>
- height, width: The video's dimensions in pixels
- title: A descriptive title
- src: The URL from Step 1.
- Add the JavaScript code. Include a
<script>
element in the body as shown in the example.- Get a reference to the iFrame's
contentWindow
using its ID. - Add an event listener to the window object for the "
message
" event. - Check if the event data is "
een-iframe-loaded
" or "een-iframe-token-expired
". - If true, send a
postMessage
to the iFrame with the required access token.
- Get a reference to the iFrame's
- Replace
<your_access_token>
with your camera's valid access token.
Example
Here is a sample code for embedding a history iFrame.
Note
Make sure to replace
<esn>
and<your_access_token>
with your camera ID and access token, respectively.
<html lang="en">
<head>
<meta charset="UTF-8">
<title>iframe example</title>
</head>
<body>
<iframe
id="test-iframe"
height="700"
width="1000"
title="History Browser"
src="https://iframe.eagleeyenetworks.com/#/history?ids=<esn>"
></iframe>
<script>
const iframe = document.getElementById("test-iframe").contentWindow;
window.addEventListener("message", event => {
if (event.data === 'een-iframe-loaded' || event.data === "een-iframe-token-expired") {
iframe.postMessage({
type: "een-token",
token: "<your_access_token>"
}, "https://iframe.eagleeyenetworks.com/");
}
});
</script>
</body>
</html>
Updated over 1 year ago